When you want to test your code that uses Typemock, you can use a Team Foundation Server Build Server. The current version of Typemock is 7.4 and this version (introduced in 6.2) has the AutoDeploy feature included. This is very important because now you can use multiple versions of Typemock on one Build server. The reason for that is that you don’t have to install Typemock on you build server anymore. To setup Typemock Autodeploy, you can use the tutorial on their help page in the chapter: Typemock Isolator Integration with Team Foundation Server 2010 (TFS). The only thing that they forgot to mention in the documentation is that there is generated a Typemock directory with a couple of dll’s in it inside your just added AutoDeploy directory. This is done after you fixed the references and restarted Visual Studio. Don’t forget to checkin that directory.
In the help documentation, they say that you have to use the workflow of Typemock to get the AutoDeploy to work. The customer that I now work for already have a custom workflow. I just created that workflow last week with some custom actions for them. So I want to integrate the custom Typemock stuff in the workflow of the customer. The customer works with TFS 2010 (just migrated a few months ago from 2008 so they have a lot of catching up to do) so this blog is aimed at TFS Build 2010. But this will also work for 2012. You can also check the documentation about Typemock with TFS 2012 on their website.
The differences between the build templates
There only 4 differences between the template that are special for Typemock. I will show them beneath. The only thing that you have watch for is the right place to put custom activities on the right place.
First open your own workflow in the designer. In your toolbox, add a new category and choose the TypeMock.TFS2010.dll assembly in the installation directory of your Typemock version on your machine. After that, three custom activities are added to your toolbox. Don’t forget to reference the dll in your project where your custom workflow is located.
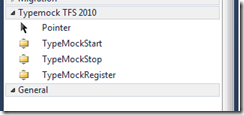
Now add the activities on the right place by dragging them into the workflow. You don’t have to edit any properties. On the left side, you see the default template of TFS 2010. On the right side the template of Typemock. Drag the activities on the same place in your own workflow.
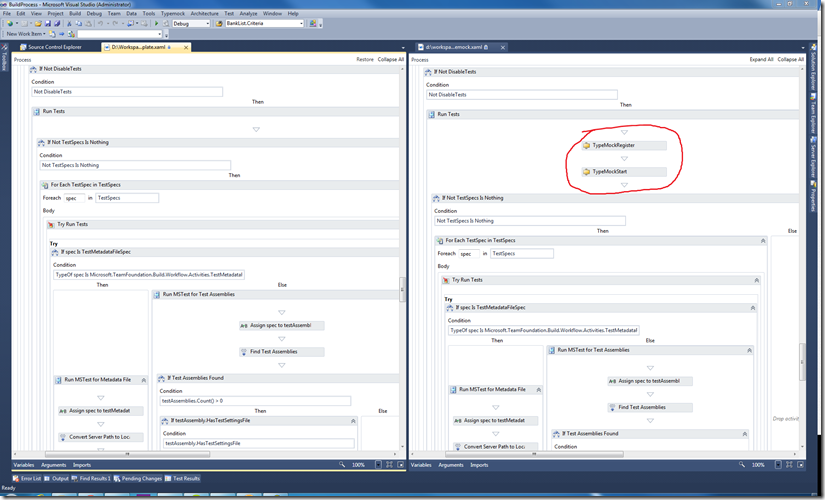
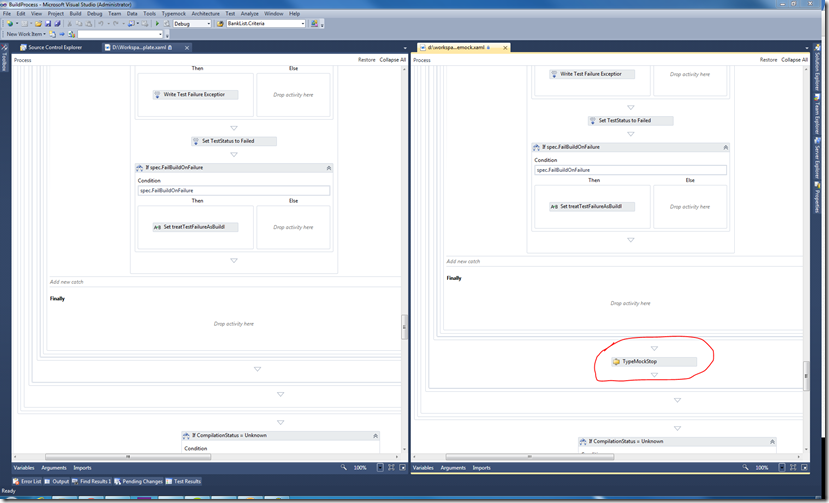
After that, add a workflow argument of the TypemockSettings type. You can optionally edit the Metadata argument to customize the text that you will see in you build definition.
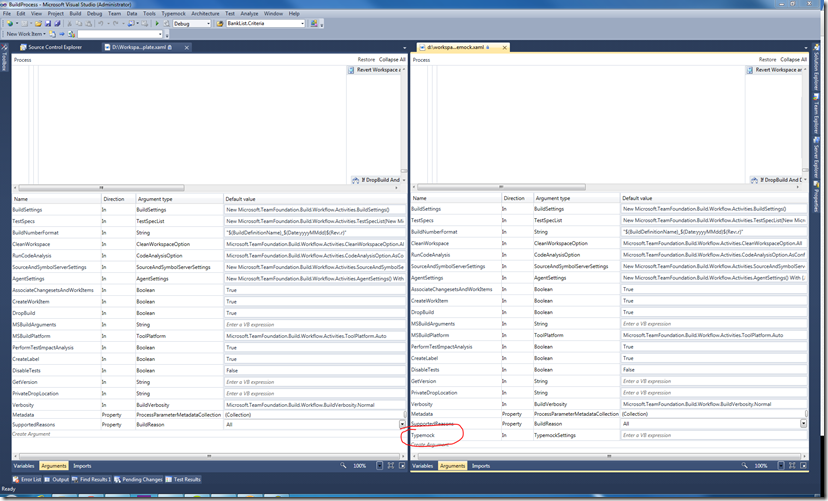
Save your workflow and checkin your workflow. Restart Visual Studio and configure the license in your build definition.
That’s it! It’s very easy. It is not more than 10 minutes of work to edit your own template. When you run your build now with the AutoDeploy feature on and with entering the right license info in your build definition, the build will succeed en will test your Typemock tests.